Addressing the Challenge of “Kysely date_trunc is not unique”
In modern web development, managing databases effectively is essential. SQL continues to be a major tool for querying and managing relational databases. With the introduction of tools like Kysely, a TypeScript SQL query builder, developers can write SQL queries more efficiently while ensuring type safety. Despite its strengths, when using advanced SQL functions like date_trunc, developers may encounter specific issues—one of which is the common challenge: “Kysely date_trunc is not unique.”
This article explores what the phrase “Kysely date_trunc is not unique” means, why it occurs, and how to resolve the problem effectively.
What is Kysely?
Kysely is a SQL query builder designed for TypeScript that allows developers to create type-safe SQL queries with ease. Unlike traditional raw SQL queries, Kysely offers an abstraction layer that makes it easier to build, manage, and scale queries. The main advantages of using Kysely include:
- Type Safety: Queries are validated during development, minimizing the risk of runtime errors.
- Flexibility: Kysely is compatible with multiple database systems such as PostgreSQL, MySQL, and SQLite.
- Developer-Friendly Syntax: The syntax is intuitive, enabling developers to construct complex queries without sacrificing performance.
Despite its numerous advantages, some operations, like using the date_trunc function, may lead to issues. One such issue is the challenge that “Kysely date_trunc is not unique.”
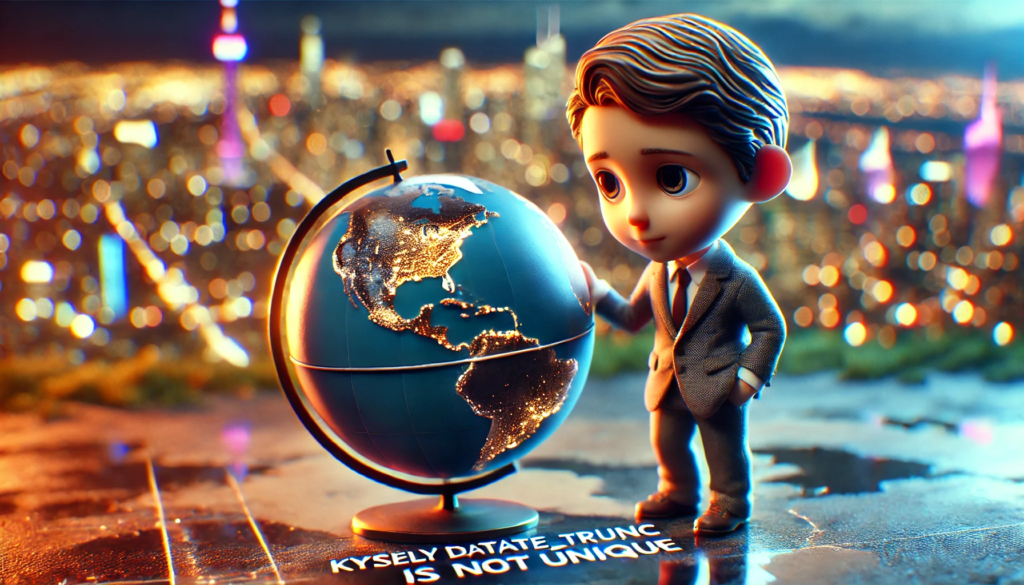
The Role of date_trunc in SQL
The date_trunc function in SQL is used to truncate timestamps to a specified precision, such as removing the hours, minutes, or seconds from a timestamp. This can be incredibly useful for data aggregation and time-based analysis. For example, if you have a timestamp with milliseconds but only need data grouped by month or day, date_trunc can simplify the process.
Example:
Imagine you have a timestamp, 2024-11-28 15:45:30.123, and you want to truncate it to the day level:
sqlCopy codeSELECT date_trunc(‘day’, ‘2024-11-28 15:45:30.123’);
— Output: 2024-11-28 00:00:00
While date_trunc is highly useful, its behavior can sometimes cause confusion when used within tools like Kysely.
Why Does the Issue “Kysely date_trunc is not unique” Occur?
The phrase “Kysely date_trunc is not unique” refers to situations where truncating dates leads to redundancy or ambiguity in the results. This problem doesn’t arise from any fault in the date_trunc function itself, but rather from how it is used and interpreted in certain scenarios.
1. Duplicate Results During Aggregation
When date_trunc is used to truncate dates and group data by these truncated values, it’s possible for multiple records to collapse into the same truncated value. For example:
sqlCopy codeSELECT date_trunc(‘month’, timestamp_column), COUNT(*)
FROM sales
GROUP BY date_trunc(‘month’, timestamp_column);
If two timestamps, 2024-11-01 and 2024-11-15, are in the database, both will truncate to 2024-11-01 00:00:00, grouping them together under the same value. This creates the illusion that the truncated value is “not unique.”
2. Conflicting Column Names in Queries
When working with Kysely, there’s a possibility that the truncated value may conflict with the name of another column or alias in your query. For example:typescriptCopy codeconst result = await db.selectFrom(‘sales’)
.select([
db.fn.dateTrunc(‘month’, ‘sales.timestamp’).as(‘month’),
‘sales.id’
])
.execute();
If there’s already a column named month in the database, this can create ambiguity, resulting in unexpected query outcomes.
3. Performance Issues Due to Indexing
Using date_trunc in queries may bypass indexes on timestamp columns since it alters the original value. This can degrade query performance as the database may not use the most efficient execution plan.
Solutions to Address the “Kysely date_trunc is not unique” Issue
To avoid the problems associated with “Kysely date_trunc is not unique”, developers can apply several best practices:
1. Use Clear Aliases for Truncated Values
Assigning explicit and unique aliases to the results of date_trunc can help avoid confusion. For example:
typescript
Copy code
const result = await db.selectFrom(‘sales’)
.select([
db.fn.dateTrunc(‘month’, ‘sales.timestamp’).as(‘truncated_month’),
db.fn.count(‘sales.id’).as(‘total_sales’)
])
.groupBy(‘truncated_month’)
.execute();
By clearly naming the truncated value (truncated_month), there’s no confusion between the original column and the derived value.
2. Precompute Truncated Values
For large datasets, you can improve performance by precomputing truncated values and storing them in a new column or a materialized view. This eliminates the need for runtime computation and ensures better query performance.
sql
Copy code
ALTER TABLE sales ADD COLUMN truncated_month TIMESTAMP;
UPDATE sales
SET truncated_month = date_trunc(‘month’, timestamp_column);
Then, in your Kysely query, you can simply reference the precomputed column:
typescript
Copy code
const result = await db.selectFrom(‘sales’)
.select([
‘truncated_month’,
db.fn.count(‘sales.id’).as(‘total_sales’)
])
.groupBy(‘truncated_month’)
.execute();
3. Index Truncated Columns
When queries frequently use date_trunc, indexing the truncated column can improve performance. This will help in efficient lookups and prevent unnecessary recalculations.
sql
Copy code
CREATE INDEX idx_sales_truncated_month ON sales (date_trunc(‘month’, timestamp_column));
4. Take Advantage of Kysely’s Type Safety
Kysely’s type-safe nature can help prevent errors due to column ambiguity. Ensure that your database schema is clearly defined and use features like autocomplete in your IDE to avoid column name conflicts.
typescript
Copy code
import { Generated, Selectable } from ‘kysely’;
interface SalesTable {
id: Generated<number>;
timestamp: string; // ISO date string
truncated_month?: string; // Optional column for precomputed values
}
const db = new Kysely<DatabaseSchema>();
By explicitly defining the schema, you reduce the chances of naming collisions during query construction.
5. Validate Results Programmatically
After executing queries that use date_trunc, it’s a good practice to validate the output to ensure the results match expectations. For instance, you can check for duplicate or unexpected values in the grouped data.
typescript
Copy code
const validateUniqueTruncations = (rows: any[]) => {
const seen: Set<string> = new Set();
for (const row of rows) {
if (seen.has(row.truncated_month)) {
throw new Error(‘Non-unique truncated month detected!’);
}
seen.add(row.truncated_month);
}
};
const rows = await query.execute();
validateUniqueTruncations(rows);
Key Facts
- What is Kysely?
- Kysely is a TypeScript-based SQL query builder designed to help developers write type-safe, efficient SQL queries. It abstracts SQL query construction while ensuring that type checks are done at compile time.
- What is the date_trunc function in SQL?
- The date_trunc function is used in SQL to truncate a timestamp to a specified level of precision, such as day, month, year, etc. It helps aggregate data based on specific time intervals.
- The Issue of Non-Unique Results in date_trunc
- The term “Kysely date_trunc is not unique” refers to situations where using date_trunc in a query results in multiple records being grouped under the same truncated value, causing the output to appear ambiguous or redundant.
- Common Causes of Non-Uniqueness
- Duplicate Timestamps: Truncating timestamps to a coarser level (like the month or day) may group multiple records under the same truncated value.
- Column Name Conflicts: When using date_trunc in Kysely, the alias for the truncated column may conflict with the name of an existing column in the database, leading to ambiguity.
- Performance Issues: Using date_trunc can bypass indexes on timestamp columns, which could lead to inefficient query execution plans.
- Solutions to Address the Issue
- Clear Aliasing: Use clear and distinct aliases for truncated values to avoid column name conflicts and ensure clarity in the query results.
- Precompute Truncated Values: For large datasets, consider precomputing truncated values in a separate column or materialized view for better performance.
- Indexing Truncated Columns: Indexing the truncated columns can improve query performance by ensuring efficient lookups.
- Utilize Kysely’s Type Safety: Leverage Kysely’s type safety features to avoid ambiguity in queries and reduce errors.
Conclusion
The issue of “Kysely date_trunc is not unique” highlights a challenge faced by developers when using the date_trunc function in SQL. This problem typically arises due to the nature of truncating timestamps, which can lead to redundancy or ambiguity in the results.
By adopting best practices such as using clear aliases, precomputing truncated values, indexing truncated columns, and leveraging Kysely’s type safety, developers can effectively resolve these challenges. Kysely remains a powerful tool for writing type-safe and efficient SQL queries, and understanding these nuances will help developers make the most of it while avoiding common pitfalls.
FAQs
Q1: What does “Kysely date_trunc is not unique” mean?
- This issue arises when using the date_trunc function in Kysely, where truncating timestamps leads to duplicate or ambiguous results. Multiple timestamps can collapse into the same truncated value, leading to the appearance of non-uniqueness.
Q2: How can I resolve the “Kysely date_trunc is not unique” issue?
- You can resolve this by using clear aliases for the truncated values, precomputing truncated values in a new column, indexing the truncated columns, and leveraging Kysely’s type safety features to avoid conflicts in your queries.
Q3: Can I avoid performance problems caused by date_trunc in Kysely?
- Yes, by precomputing truncated values and indexing those columns, you can improve performance and avoid issues related to inefficient query execution plans.
Q4: Does date_trunc in SQL affect the underlying data?
- No, the date_trunc function only affects how the timestamp is displayed in the query result. It does not modify the underlying data in the database unless explicitly updated with new values.
Q5: Why is it important to use aliases when working with date_trunc in Kysely?
- Using aliases is crucial to avoid confusion or conflicts with existing column names in the database. This ensures that the query is clear and that the results are correctly interpreted.
Q6: How does Kysely help in managing SQL queries with date_trunc?
- Kysely provides type-safe query construction, which helps avoid common errors like column conflicts and ensures that the query is correctly validated during development, reducing runtime errors.
Q7: How do I handle duplicate results when using date_trunc for data aggregation?
- To handle duplicate results, you can group the truncated values correctly and ensure that unique truncations are identified using techniques such as validation or precomputing values.
Stay in touch to get more news & updates on Live Hint