JAX is an advanced numerical computing framework widely used for high-performance machine learning and scientific computations. Its ability to handle automatic differentiation seamlessly and execute operations on GPUs and TPUs makes it an ideal choice for researchers and developers. One of the key features of JAX is its functional programming paradigm, which incorporates constructs like loop carry, alongside operations such as arange. This article delves into how the jax.numpy.arange function works in conjunction with loop carry, providing both conceptual insights and practical examples.
Overview of jax.numpy.arange?
jax.numpy.arange is the JAX equivalent of the NumPy arange function. It creates a sequence of numbers within a specified range, with evenly spaced values. The function follows a simple syntax:
jax.numpy.arange(start, stop, step)
Parameters:
- start: The initial value of the sequence (inclusive).
- stop: The final value of the sequence (exclusive).
- step: The increment between consecutive values (default is 1).
Example:
import jax.numpy as jnp
# Generate a sequence from 0 to 10 (exclusive) with a step of 2
sequence = jnp.arange(0, 10, 2)
print(sequence) # Output: [0, 2, 4, 6, 8]
arange is especially useful in operations involving tensors, defining input ranges, or iterating over a series of indices.
Exploring the Concept of Loop Carry in JAX
In JAX, the term “loop carry” refers to the concept of preserving the state between iterations of a loop. Unlike traditional imperative programming, JAX utilizes functional programming, which avoids mutable states and side effects. Functions like jax.lax.scan and jax.lax.while_loop supports loop operations, with the carry representing variables that retain their values across iterations.
Key Constructs:
- jax.lax.scan: Ideal for iterative computations over sequences, this function returns both the final state and intermediate results.
- jax.lax.while_loop: Facilitates loops with specific conditions and a carry state. It is optimized for scenarios where the number of iterations depends on dynamic conditions.
Both of these constructs are JIT-compiled for performance and support seamless gradient computation.
Example of Loop Carry in Action:
import jax
# Define a looping function
@jax.jit
def loop_example():
def body_fn(carry, x):
carry = carry + x # Update the carry value
return carry, carry # Return both updated carry and result
values = jnp.arange(5) # [0, 1, 2, 3, 4]
initial_carry = 0 # Starting value for the carry
final_carry, outputs = jax.lax.scan(body_fn, initial_carry, values)
return final_carry, outputs
final_carry, outputs = loop_example()
print(final_carry) # Final carry: 10
print(outputs) # Outputs: [0, 1, 3, 6, 10]
In this example, the body_fn function describes how the carry evolves with each loop iteration, and jax.lax.scan handles the looping mechanism automatically.
Leveraging arange and Loop Carry Together for Efficient Iterations
By combining arange with loop carry, JAX enables efficient and flexible iterative computations over ranges of values. Below are some common use cases for this powerful combination:
1. Making Sum of a Sequence
Using arange together with loop carry, you can compute cumulative sums with ease:
@jax.jit
def cumulative_sum():
def body_fn(carry, x):
carry += x # Add x to the carry
return carry, carry
values = jnp.arange(1, 6) # [1, 2, 3, 4, 5]
initial_carry = 0
final_carry, outputs = jax.lax.scan(body_fn, initial_carry, values)
return final_carry, outputs
final_sum, cumulative_sums = cumulative_sum()
print(final_sum) # Output: 15
print(cumulative_sums) # Output: [1, 3, 6, 10, 15]
2. Fibonacci Sequence Calculation
Loop carry can be applied to generate sequences like the Fibonacci series:
@jax.jit
def fibonacci(n):
def body_fn(carry, _):
a, b = carry
return (b, a + b), b
initial_carry = (0, 1) # Starting values for Fibonacci
_, outputs = jax.lax.scan(body_fn, initial_carry, jnp.arange(n))
return outputs
print(fibonacci(10)) # Output: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55]
3. Iterative Gradient Descent Optimization
Loop carry is also useful for performing gradient descent updates in an iterative manner:
@jax.jit
def gradient_descent_step(weights, gradient):
learning_rate = 0.01
return weights – learning_rate * gradient
@jax.jit
def gradient_descent_loop(initial_weights, gradients):
def body_fn(carry, grad):
updated_weights = gradient_descent_step(carry, grad)
return updated_weights, updated_weights
final_weights, history = jax.lax.scan(body_fn, initial_weights, gradients)
return final_weights, history
weights = jnp.array([0.5, 0.5])
gradients = jnp.array([[0.1, 0.2], [0.2, 0.1], [0.3, 0.4]])
final_weights, weight_history = gradient_descent_loop(weights, gradients)
print(final_weights) # Updated weights after all steps
print(weight_history) # History of weights at each step
4. Managing Multi-Dimensional Computations
Loop carry can be adapted to handle more complex states in multi-dimensional computations:
@jax.jit
def multi_dim_loop(initial_state, values):
def body_fn(carry, val):
carry = carry * val # Custom operation
return carry, carry
final_state, results = jax.lax.scan(body_fn, initial_state, values)
return final_state, results
initial = jnp.array([1.0, 1.0])
inputs = jnp.array([[1.1, 1.2], [0.9, 0.8], [1.5, 1.3]])
final_state, computations = multi_dim_loop(initial, inputs)
print(final_state)
print(computations)
Benefits of Using JAX for Loop Carry Operations
- Performance: JAX optimizes loops by compiling them for execution on GPUs and TPUs, ensuring high performance.
- Automatic Differentiation: JAX can compute gradients of loop-based operations without additional effort.
- Functional Approach: By adhering to a functional programming paradigm, JAX avoids mutable states and side effects, enhancing modularity and reproducibility.
- Scalability: JAX can handle large-scale computations and data effortlessly.
Best Practices for Working with JAX and Loop Carry
- Utilize JIT Compilation: To maximize performance, always use @jax.jit for your loop functions.
- Minimize Carry State Size: Reducing the carry state size improves memory and computational efficiency.
- Optimize arange: Ensure that the parameters of arange are set appropriately for the problem to avoid unnecessary computation.
- Test with Smaller Inputs: Begin debugging with smaller datasets before scaling up to larger inputs.
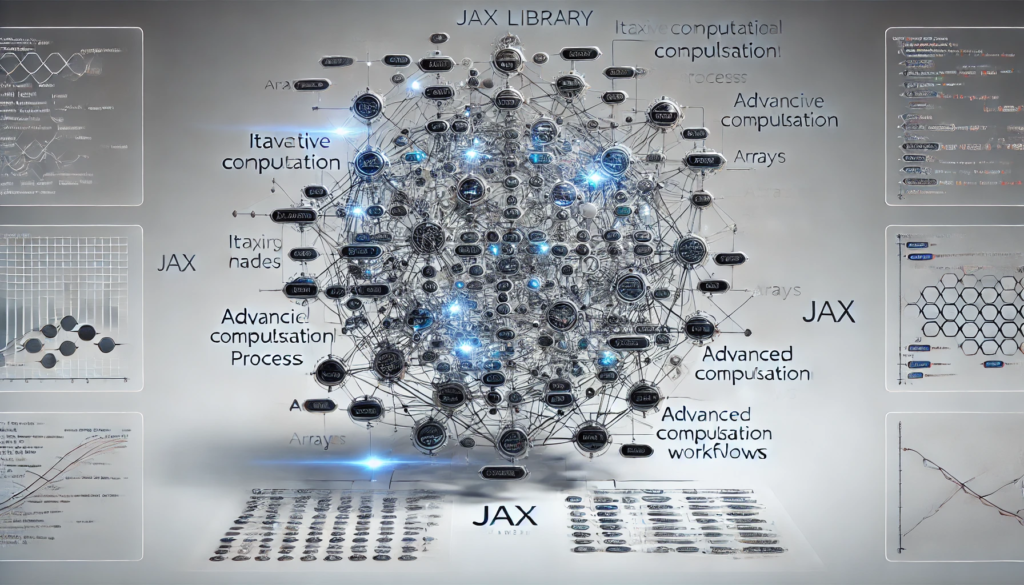
Key Facts about Loop Carry:
- State Preservation Across Iterations:
Loop Carry in JAX is used to preserve and update state values between iterations in a loop. This allows variables to evolve over time and maintain information, unlike traditional loops that only deal with local variables during each iteration.
- Functional Programming Paradigm:
JAX utilizes a functional programming approach, where operations like Loop Carry are designed to avoid mutable states and side effects. This enhances reproducibility and modularity in computations.
- Optimized for High-Performance Computing:
Loop Carry operations in JAX are JIT (Just-In-Time) compiled, meaning they are optimized for execution on accelerators like GPUs and TPUs. This ensures efficient performance for iterative and large-scale computations.
- Support for Automatic Differentiation:
One of the major advantages of using Loop Carry in JAX is its seamless integration with JAX’s automatic differentiation system. You can compute gradients through iterative operations like Loop Carry without additional manual configuration.
- Works with jax.lax.scan and jax.lax.while_loop:
JAX offers constructs like jax.lax.scan and jax.lax.while_loop to implement Loop Carry in iterative operations. These constructs help manage loop conditions and carry states efficiently, providing a functional alternative to traditional loops.
Final Thoughts on JAX arange and Loop Carry
The combination of arange and loop carry in JAX presents an efficient and functional approach to implementing iterative algorithms. Whether you’re calculating cumulative sums, generating Fibonacci sequences, or performing gradient descent, these tools streamline the development process. By leveraging JAX’s features, you can build scalable, high-performance solutions for a wide variety of computational tasks.
FAQs about Loop Carry:
1. What is Loop Carry in JAX?
Loop Carry in JAX refers to the preservation of state across iterations in a loop. It allows variables to carry forward their updated values, which is essential in iterative computations, such as summing a sequence or generating Fibonacci numbers. JAX achieves this in a functional programming style, which avoids side effects and mutable states.
2. How does Loop Carry differ from traditional loops?
In traditional imperative programming, loop variables are typically reinitialized in each iteration. With Loop Carry in JAX, however, the variable (carry) is updated and passed along to the next iteration, enabling more complex operations and maintaining continuity across iterations.
3. Which functions in JAX are used to implement Loop Carry?
JAX provides functions like jax.lax.scan and jax.lax.while_loop to implement Loop Carry. These functions allow you to specify a carry state that evolves during each iteration, while also handling the loop condition and terminating based on dynamic criteria.
4. Can Loop Carry be used with automatic differentiation in JAX?
Yes, Loop Carry works seamlessly with JAX’s automatic differentiation system. You can compute gradients of functions that involve iterative loops with carry states, enabling efficient gradient-based optimization in tasks like gradient descent.
5. What are the benefits of using Loop Carry in JAX for machine learning?
Using Loop Carry in JAX for machine learning provides several advantages: it supports high-performance execution on GPUs/TPUs, simplifies the implementation of iterative algorithms, and allows for more efficient gradient calculations during optimization processes. Additionally, its functional nature reduces the chances of errors from mutable states, making models more robust and reproducible.
Stay in touch to get more news & updates on Live Hint